
This tutorial shows you how to encode and decode characters with UTF-8, UTF-16, and UTF32 in Dart.
Character encoding is used to represent a character as bytes. There are some encoding formats, UTF-8 is the most commonly used. There are also other UTF encodings such as UTF-16 and UTF-32. The main diference is how many bytes needed to represent a character. UTF-8 uses at least one byte, UTF-16 uses at least 2 bytes, while UTF-32 always uses 4 bytes. This tutorial gives you examples of how to perform encoding and decoding with those formats in Dart, including how to set endianness and BOM (Byte Order Mark). Google arts and culture website.
Dependencies
- Nov 23, 2019 There are some encoding formats, UTF-8 is the most commonly used. There are also other UTF encodings such as UTF-16 and UTF-32. The main diference is how many bytes needed to represent a character. UTF-8 uses at least one byte, UTF-16 uses at least 2 bytes, while UTF-32 always uses 4 bytes.
- UTF-8 is a Unicode encoding that represents each code point as a sequence of one to four bytes. Unlike the UTF-16 and UTF-32 encodings, the UTF-8 encoding does not require 'endianness'; the encoding scheme is the same regardless of whether the processor is big-endian or little-endian. UTF8Encoding corresponds to the Windows code page 65001.
- Web developer and programmer tools World's simplest UTF8 encoder. Just paste your text in the form below, press UTF8 Encode button, and you get UTF8-encoded data. Press button, get UTF8.
A Utf8Codec encodes strings to utf-8 code units (bytes) and decodes UTF-8 code units to strings. The utf8encode function encodes an ISO-8859-1 string to UTF-8. Unicode is a universal standard, and has been developed to describe all possible characters of all languages plus a lot of symbols with one unique number for each character/symbol. However, it is not always possible to transfer a Unicode character to another computer reliably.
Dart's built-in convert
package only supports UTF-8. For UTF-16 and UTF-32, you can use utf
package. Add the following in the dependencies
section of your pubspec.yaml
file, then run `Get dependencies'.
Using convert
Package
To use Dart's convert
package, import the library first by adding the following:
To perform encoding, use:
You only need to pass the string to be encoded.
To decode the bytes into a String, use:
If allowMalformed
is set to true
, it will replace invalid or unterminated octet sequences with the Unicode Replacement character `U+FFFD` (�). Connect ipad to lg tv. If it's set to false
and invalid sequence exist, it will throw FormatException
.
Here is the usage example:
Output:
Using utf
Package
Besides UTF-8, the utf
also supports UTF-16 and UTF-32. Below are the list of functions for encoding and decoding provided by utf
package.
The functions for different encodings are similar. For encoding, the only required parameters is the value (String). For UTF-16 and UTF-32, you can choose between BE (Big Endian) and LE (Little Endian) by using function with be
and le
suffix respectively. By default, the functions without suffix uses Big Endian. The functions with suffix are not available for UTF-8 as it's read byte by byte regarless of the CPU architecture. For functions with BE and LE suffix, there is also an optional parameter:
writeBOM
: determines whether the BOM (Byte Order Mark) should be written.
For decoding, UTF-16 and UTF-32 also have the BE and LE variants. You need to pass the bytes (List
) as the first argument. The optional parameters are:
offset
: an offset into a list of bytes.length
: limit the length of the values to be decoded.stripBom
: whether to strip the leading BOM.replacementCodepoint
: the replacement character. Default to0xffd
.
Below are the examples of using the encoding functions mentioned above on the same string as well as the functions for decoding, followed by the output.
Output::
You can see the difference ouput bytes as the result of using different encodings and endiannesses. For decoding, using the right function based on the encoding and endianness is also important to get the correct value.
Farming Simulator 19 expands even further on March 16 with the Rottne DLC! Available for PC and Mac, this new pack from one of the industry's leading forestry equipment. The forest calls you in the upcoming Rottne DLC on March 16! Tue, March 9, 2021 3:25 AM PST.

R utf8_encode
Escape the strings in a character object, optionally adding quotes or spaces, adjusting the width for display.
utf8_encode is located in package utf8. Please install and load package utf8 before use.
Encoder
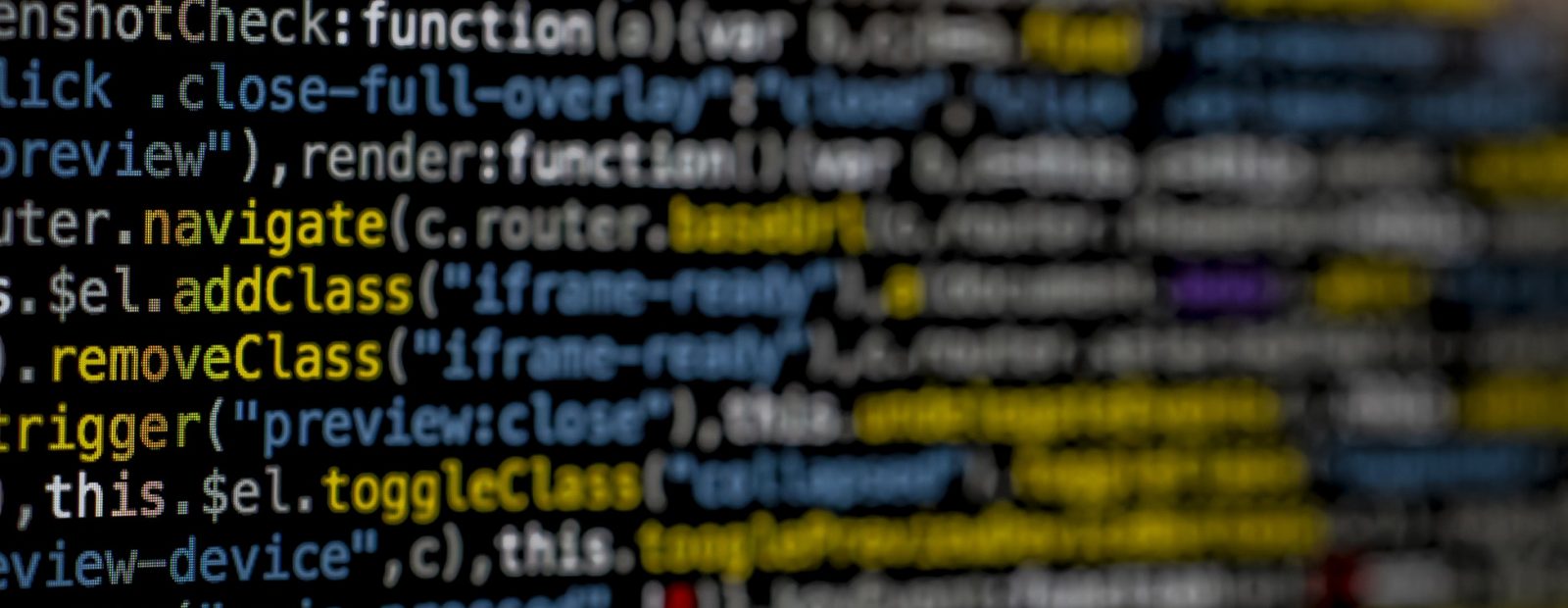
This tutorial shows you how to encode and decode characters with UTF-8, UTF-16, and UTF32 in Dart.
Character encoding is used to represent a character as bytes. There are some encoding formats, UTF-8 is the most commonly used. There are also other UTF encodings such as UTF-16 and UTF-32. The main diference is how many bytes needed to represent a character. UTF-8 uses at least one byte, UTF-16 uses at least 2 bytes, while UTF-32 always uses 4 bytes. This tutorial gives you examples of how to perform encoding and decoding with those formats in Dart, including how to set endianness and BOM (Byte Order Mark). Google arts and culture website.
Dependencies
- Nov 23, 2019 There are some encoding formats, UTF-8 is the most commonly used. There are also other UTF encodings such as UTF-16 and UTF-32. The main diference is how many bytes needed to represent a character. UTF-8 uses at least one byte, UTF-16 uses at least 2 bytes, while UTF-32 always uses 4 bytes.
- UTF-8 is a Unicode encoding that represents each code point as a sequence of one to four bytes. Unlike the UTF-16 and UTF-32 encodings, the UTF-8 encoding does not require 'endianness'; the encoding scheme is the same regardless of whether the processor is big-endian or little-endian. UTF8Encoding corresponds to the Windows code page 65001.
- Web developer and programmer tools World's simplest UTF8 encoder. Just paste your text in the form below, press UTF8 Encode button, and you get UTF8-encoded data. Press button, get UTF8.
A Utf8Codec encodes strings to utf-8 code units (bytes) and decodes UTF-8 code units to strings. The utf8encode function encodes an ISO-8859-1 string to UTF-8. Unicode is a universal standard, and has been developed to describe all possible characters of all languages plus a lot of symbols with one unique number for each character/symbol. However, it is not always possible to transfer a Unicode character to another computer reliably.
Dart's built-in convert
package only supports UTF-8. For UTF-16 and UTF-32, you can use utf
package. Add the following in the dependencies
section of your pubspec.yaml
file, then run `Get dependencies'.
Using convert
Package
To use Dart's convert
package, import the library first by adding the following:
To perform encoding, use:
You only need to pass the string to be encoded.
To decode the bytes into a String, use:
If allowMalformed
is set to true
, it will replace invalid or unterminated octet sequences with the Unicode Replacement character `U+FFFD` (�). Connect ipad to lg tv. If it's set to false
and invalid sequence exist, it will throw FormatException
.
Here is the usage example:
Output:
Using utf
Package
Besides UTF-8, the utf
also supports UTF-16 and UTF-32. Below are the list of functions for encoding and decoding provided by utf
package.
The functions for different encodings are similar. For encoding, the only required parameters is the value (String). For UTF-16 and UTF-32, you can choose between BE (Big Endian) and LE (Little Endian) by using function with be
and le
suffix respectively. By default, the functions without suffix uses Big Endian. The functions with suffix are not available for UTF-8 as it's read byte by byte regarless of the CPU architecture. For functions with BE and LE suffix, there is also an optional parameter:
writeBOM
: determines whether the BOM (Byte Order Mark) should be written.
For decoding, UTF-16 and UTF-32 also have the BE and LE variants. You need to pass the bytes (List
) as the first argument. The optional parameters are:
offset
: an offset into a list of bytes.length
: limit the length of the values to be decoded.stripBom
: whether to strip the leading BOM.replacementCodepoint
: the replacement character. Default to0xffd
.
Below are the examples of using the encoding functions mentioned above on the same string as well as the functions for decoding, followed by the output.
Output::
You can see the difference ouput bytes as the result of using different encodings and endiannesses. For decoding, using the right function based on the encoding and endianness is also important to get the correct value.
Farming Simulator 19 expands even further on March 16 with the Rottne DLC! Available for PC and Mac, this new pack from one of the industry's leading forestry equipment. The forest calls you in the upcoming Rottne DLC on March 16! Tue, March 9, 2021 3:25 AM PST. Dec 08, 2020 Season Pass Expand your Farming Simulator 19 experience with the Season Pass, including the following content: Alpine Farming Expansion, Platinum Expansion, Kverneland & Vicon Equipment Pack, Bourgault DLC, John Deere Cotton DLC, Anderson Group Equipment Pack Customers who bought this item also bought. Expand your Farming Simulator 19 experience with the Season Pass, including the following content - Alpine Farming Expansion - Platinum Expansion - Rottne DLC - GRIMME Equipment Pack - Kverneland Vicon Equipment Pack - Bourgault DLC - John Deere Cotton DLC - Anderson Group Equipment Pack Save money on content for this game by purchasing the Season Pass. Save money on content for this game by purchasing the Season Pass. Expand your Farming Simulator 19 experience with the Season Pass, granting you access to current and future DLC packs. Make sure to secure yours right now!
R utf8_encode
Escape the strings in a character object, optionally adding quotes or spaces, adjusting the width for display.
utf8_encode is located in package utf8. Please install and load package utf8 before use.
Encoder
Utf8_encode
Utf8_encode In Php
Return Values: A character object with the same attributes as x but with Encoding set to 'UTF-8'.
Details:utf8_encode encodes a character object for printing on a UTF-8 device by escaping controls characters and other non-printable characters. When display = TRUE, the function optimizes the encoding for display by removing default ignorable characters (soft hyphens, zero-width spaces, etc.) and placing zero-width spaces after wide emoji. When output_utf8() is FALSE the function escapes all non-ASCII characters and gives the same results on all platforms.
See Also:utf8_print.